CS 102 Lab # 1
An Introduction to Java Programming
Objective: To demonstrate the use of Dr. Java
In order to create the folders and programs you need for the lab, download this file by right-clicking on the link; click "Save Link As " in the dialogue window. Save the file in your home folder.
We will need to use terminals throughout the labs. To add the terminal icon to the desktop, first get to the application by clicking "Applications->Accessories". Right click on the "Terminal" icon and select "Add this launcher to desktop". Now you have the Terminal icon on the desktop. You can just double click it to open a terminal window.
Now open a terminal window. In the new window that opens, type source ./init and press Enter. Now log out and log back in again to make sure your environment is properly set up. Start the browser again using the globe icon to view the lab online.
For this
lab you'll construct a Java program to draw the face of a robot, as shown below.
You will use a program named Dr. Java which provides
everything you need to type in and run Java programs. Here is how to use Dr. Java:
Explain
There should
be an icon on your desktop for Dr. Java; Double-click it to run the
program. You should see a window much like this:
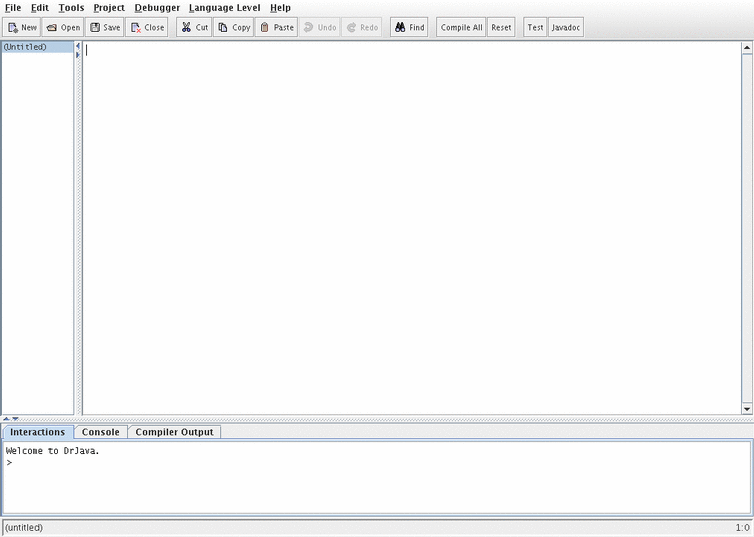
You will accomplish the assignment by adding code to an existing file that
we have provided for you. Here is what you should do:
Explain
You are going to create your program by beginning with a skeleton that
we have provided for you. In your home directory (labeled by your username), create a CS102 directory. In side the CS102 directory, create a subdirectory lab1. Download the file Robot.java by clicking it while holding
the shift key.
In order to
begin working with it you must
open it with Dr. Java.
From
the "File" menu, select "Open", or use the Control-O
keyboard shortcut.
A dialogue window should appear:
Navigate to the CS102/lab1 folder and
double click Robot.java.
Now the Dr. Java window should
look like this:
The large area on the right side of the screen displays the contents
of the "Robot.java" file. The text of the program as it
should initially appear is shown below
import objectdraw.*;
// CS 102 Laboratory 1 --- practice program
// Enter your name, lab section and the date here.
public class Robot extends FrameWindowController{
public void begin()
{
// commands placed here are executed when the program begins to execute
}
public void onMouseEnter(Location point)
{
// commands placed here are executed when the mouse enters the program window
}
public void onMouseExit(Location point)
{
// commands placed here are executed when the mouse leaves the program window
}
public void onMousePress(Location point)
{
// commands placed here are executed when the mouse button is depressed
}
public void onMouseRelease(Location point)
{
// commands placed here are executed when the mouse button is released
}
}
The text we have placed in
"Robot.java" is the skeleton of a complete Java program. It
includes the header for the definition of a class
Robot
that
extends
FrameWindowController and within the class, headers
for
the
event handling methods you will use. We have not, however, included
any Java commands within the bodies of these methods, only a Java
comment that reminds you when the Java system will follow any
instructions you might add to the method body.
You should follow our lead and always begin writing your programs by
typing
comments rather than actual Java commands. Near the top of the file
we have included a temporary comment telling you to enter your name,
lab section time and the current date.
Do this now.
Dr. Java provides the familiar
capabilities of a typical word processor or text editor. You can
position the cursor using the mouse or arrow keys. You can enter text
and/or cut and paste text using items in the edit menu.
Use these
features now to replace our comment with your name and other
information.
Next, add Java
instructions to the body of the begin
method that will draw the face of a robot. This is the
first method skeleton we have included in "Robot.java".
The first thing to do is
to replace our comment
// commands placed here are executed when the program begins to execute
with something that describes what you intend the method to do, like
// draws the face of a robot when the program begins to execute
You should get in the habit of updating comments to keep them as
accurate as possible as you add instructions to your programs. Not
only does this mean you will not have to go back and add comments when
you're done, it will help you to remember what everything is supposed
to do as you
are writing your programs.
As a
first step, let's add the
single instruction needed to draw the
rectangle that frames the face. The form of the
command you will need to enter is:
new FramedRect(x, y, width, height, canvas);
where
x,y,width, and
height need to be replaced by the numbers describing the
position and size of the rectangle. This line should be placed
immediately after the comment line in
begin (i.e.,
just
before the line containing the "}" that ends the method body).
Type in the values for the
x,y,width, and height of the rectangle.
Adjust the values to get a proper size and proportion.
Now you are ready to
compile and run your program and
see if it
works.
- To compile, click the Compile button on the tool bar. Alternatively, you can use Function Key 5 if
you like. The first time you do this, Dr. Java may ask you if you
want to save first. If so, click "Yes". You can also check
the box in the dialogue to automatically save in the future.
- Important: During this process, Dr. Java may encounter one or more
grammatical or typographical errors in your program. If errors are
discovered, Dr. Java will abort the process, and display error
messages in the bottom section of its window. The first error and
the corresponding line in your program will be highlighted.
Unfortunately, these error message are
not always very clear! If you cannot decipher the error messages you
receive, ask me for help. You will get better at
understanding error messages as the semester progresses.
Since Dr. Java really doesn't know how to correct your errors, a
single error can leave it sufficiently confused that it reports many
bizarre errors. Always start by correcting the first error
reported. If the remaining errors don't seem to make sense, they
probably don't. They are just a result of
Dr. Java's confusion. Remember,
fix the first error, then compile again.
- Once you get the message
"Last
compilation completed successfully.
", you can run the
program. Click the mouse on the Interactions tab at the lower
left. You should see something like this in the pane:
- Type the following exactly as it appears, including the
semicolon:
new Robot();
- A new window should appear - this is your program's window. A rectangle
should appear because when the Java program begins to execute, your begin method is invoked,
which
includes the instruction to construct a rectangle. If this happens,
smile. If not, press the "File" menu item and select
"Quit" to leave your program.
Examine the instructions you typed
carefully to see if you can find any mistakes. If so, correct them
and select "Run" again. Otherwise, ask for help.
When you run your program, it starts up a new
program that is separate from Dr. Java. While this program is
running, you can return to Dr. Java by just clicking on any portion
of a Dr. Java window that is visible on your screen. However, to
avoid confusion, it is a good idea to "Quit" the old copy of your
program that Dr. Java created before running it again.
Once your rectangle program is working, you should add more
instructions to draw a complete warning sign.
Back in Dr. Java,
immediately beneath the line you added to draw the rectangle, add
all the other components.
Each time you add
a line, compile
again (function key F5) to see if the program is still correct.
You might
find it convenient to look at the objectdraw
cheat sheet to remind you
about the names of the objects and method to use.
When you are done, your program should draw the face of a robot, and then do nothing until you tell it to quit.
Finally, you need to make your program responsive to mouse events. Here is what you need to do:
Explain
Now, to
explore event handling methods a bit more, revise your program so that
it reacts to the mouse in more interesting ways. In the revised
version, the face will change as the program runs. In
particular, when the user moves the mouse into the program window or
presses the mouse, your revised code will alter the appearance of the
face.
Note that the order of
instructions within a method is important, but the order among different methods in your program is not. Only the
names of the methods are important (though we urge you to put them in a logical order
so that it will be easier for all of us to read!).
Making the face change in response to the mouse is a bit trickier.
There is a setColor operation that you can use to
change the color of the components. There is also an obvious place to tell
Java to make this change. The onMouseEnter method is executed
whenever the mouse moves into your
program window. Placing an appropriate setColor in that
method
would do the trick.
The problem is that you can't simply say setColor in the
onMouseEnter method. If that was all you said, Java has no way
of
knowing which of the several objects' color to change. It could
change the rectangle, the oval, the text, or all of them, etc. Your code
has to be more specific and identify the object that should change.
To be able to specify the object whose color we
wish to set, we will have to give it a name. You can use names such as
leftEye, rightEye, message, or any name that seems appropriate. However, keep in mind that any name you come up with must be meaningful, but only to yourself but also to other readers. Names such as abc and 123 are considered bad names and should be avoided.
Important: Like for any natural language such as English, style are very important for a programming language. There are certain style conventions and naming conventions that must be following. Failing to follow these rules will result in losing points.
Associating a name with an object requires two steps. First, we have
to include a line that declares or "introduces" the name. This line
informs Java that we plan to use a particular name within the program.
At this point, we don't need to tell Java what object it should be
associated with, but we do need to specify what sort of object it will
eventually be associated with. We plan to associate the name message
with an object created as new Text, so we have to tell
Java that the name will be associated with a Text object. The
form of a Java declaration is simply the name being declared preceded
by the type of object with which it will be associated. So, the form
for our declaration is:
Text message;

You
should add a line containing
this declaration to your program
immediately
before the heading of the
onMouseClick
method.
Now you have to tell Java which object to associate with the name
message. The following line in your begin method does just that:
message = new Text("TRANSMITTING", ..., canvas);
This is an example of a construct called an
assignment
statement. It tells Java that in addition to creating the new
object,
it should associate a name with it. Shortly, you will convert some of
your other constructors into assignments. Consider the following question: Why is this line belong to the
begin method, instead of
the
onMousePress method?
Now that the text has a name, we can use the name to change its
color.
Within
the body of the begin method add the line:
message.setColor(Color.red);
Then, run your program (correcting any errors as needed).
Next we need to hind message when the program starts, and show it when the mouse is pressed. This can be accomplished by adding the following line to the begin method:
message.hide();
and the following line to the onMousePress method:
message.show();
You should be able to figure out
what to add to make it
disappear again when the mouse is released. Give it a try.
To get more practice using names and other event handling methods,
we
would like you to modify your program a bit more.
Complete your program so that it behaves exactly like the sample program given in the beginning of the page.
You may find the objectdraw
cheat sheet helpful.
This lab is worth 20 points, which are distributed as
follows:
Value |
Feature |
6 pts. |
Drawing the face correctly when the
program starts (it does not have to be exactly the same as the sample program, but it should look similar and be symmetric) |
6 pts. |
React to mouse events in the same way as the sample program |
4 pts. |
Meaningful names used in
declarations |
4 pts. |
Good and consistent formatting |